Reflex: React.js in Python!
Introduction
Hello Pythonologists!
This post is about Reflex, a React.js based python web app framework using Chakra UI and a short tutorial on making a random quote generator using Reflex. You can find more information on reflex.dev. I have also written about 4 Python web frameworks in another post.
What is Reflex Framework?
Reflex is a web framework that allows you to create web apps in pure Python. You don’t need to write any HTML, CSS (maybe a bit), or JavaScript code. Reflex handles everything for you, from the frontend to the backend.
In this tutorial, I will show you how to make a simple web app that displays a random quote when you click on a button. We will use a simple Quote API to get the quotes and the Reflex framework to create the following app.
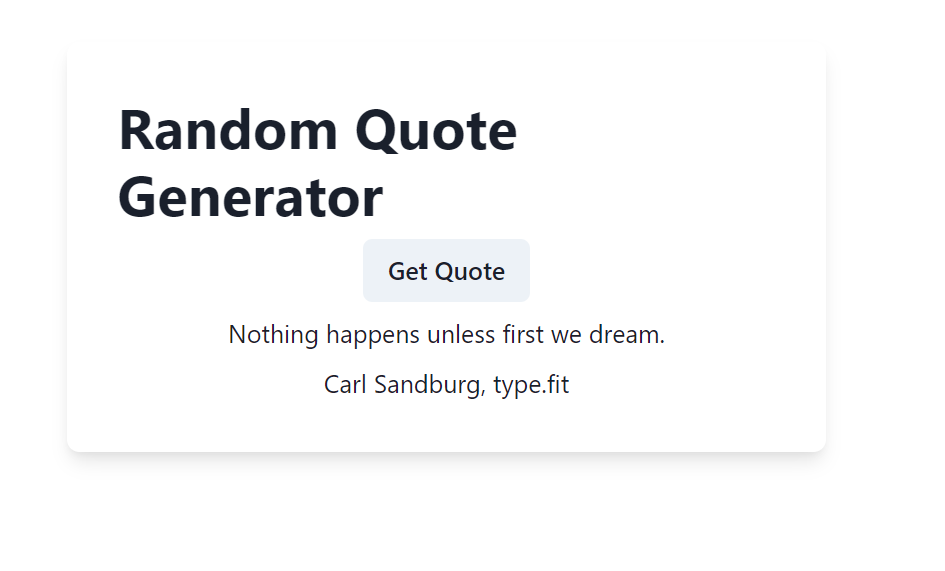
To follow this tutorial, you need to have Python 3.7+ and Node.js 16.8.0+ installed on your machine. You also need to install Reflex using pip:
pip install reflex
Once you have installed Reflex, you can create a new project using the reflex command line tool:
mkdir quote-app
cd quote-app
reflex init
This will create a template app in your quote-app directory. You can run this app in development mode using:
reflex run
You should see your app running at http://localhost:3000.
Now, let’s modify the source code in quote-app/quote-app.py to make our random quote generator. First, we need to import the requests module to make API calls and the Reflex framework:
# first install requests by 'pip install requests'
import requests
# we give reflex an alias of rx
import reflex as rx
# in order to get some random quotes
import random
Next, we need to define a state class that will store the quote data. We will use the rx.State to make our class reactive, meaning that any changes in the state will automatically update the UI:
class QuoteState(rx.State):
"""The app state."""
quote :str = ""
author :str = ""
def get_quote(self):
"""Get a random quote."""
response = requests.get("https://type.fit/api/quotes")
data = response.json()
random_number :int = random.randint(0, 10)
self.quote = data[random_number]['text']
self.author = data[random_number]['author']
Next, we need to define a function that will return the UI of our app. The function returns Reflex components: rx.center() which is used as a container, centers its child elements. The rx.vstack() component makes sure that the elements in it are vertically stacked. rx.heading(), rx.text(), and rx.button() are self-explanatory. Inside the button we have an on_click event, which runs the get_quote method in our QuoteState class, meaning that it will fetch a new random quote from the api and assigns the quote and author to same values. You can also see that some styling has been used as props (padding, shadow, border_radius). You can virtually use all css properties as props.
def home():
return rx.center(
rx.vstack(
rx.heading("Random Quote Generator"),
rx.button("Get Quote", on_click=QuoteState.get_quote),
rx.text(QuoteState.quote),
rx.text(QuoteState.author),
padding="2em",
shadow="lg",
border_radius="lg",
),
width="100%",
height="100vh",
)
Finally, we need to create an app instance and add our state and page to it. We also need to compile the app using the app.compile() method:
app = rx.App()
app.add_page(home, title="Random Quote Generator")
app.compile()
That’s it! We have created our random quote generator web app using Reflex. You can run it again using:
reflex run
You should see something like this:
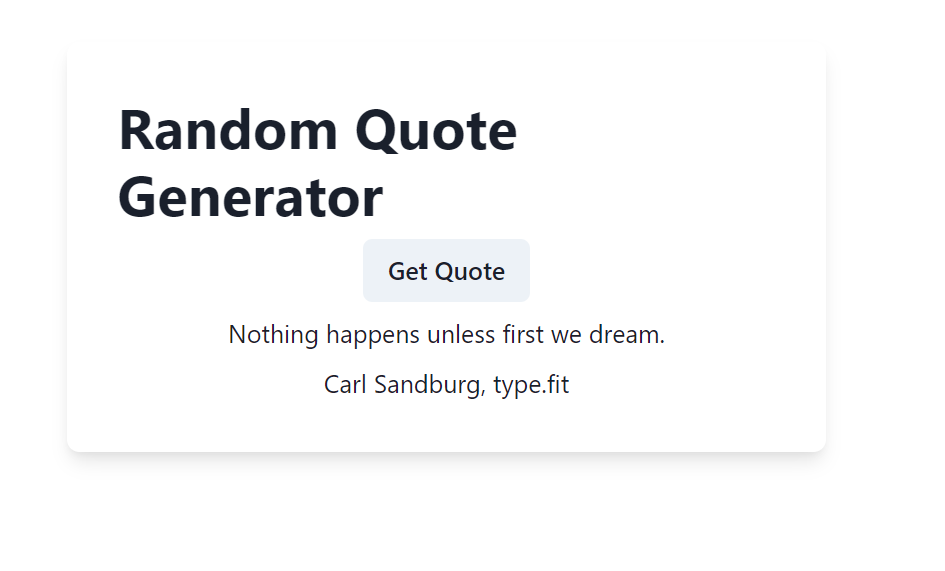
You can click on the button to get another random quote.
I hope you enjoyed this tutorial and learned something new about Reflex. If you want to learn more about Reflex, you can visit their website, read their docs, or check out their GitHub repository. Happy coding!
Here is a video for this tutorial on my Youtube channel