Introduction
If you’re a Python enthusiast or if you do text analytics and often find yourself working with a Portable Document Format file known as a PDF file, you’ll want to take a close look at the following Python PDF libraries. I have prepared a list of the most powerful and popular Python libraries for working with PDF files. The first one (IronPDF) is the most powerful PDF library on the list and is NOT free (it has a free 30-days trial period), but the rest are free with their own limitations.
- IronPDF: A powerful library brought to you by Iron Software. What sets IronPDF apart from other free PDF libraries is robustness and versatility in creating PDFs from HTML, URLS, Images…and powerful capabilities to format and edit PDFs.
- PyPDF: This is a pure Python PDF library that can be used to read and write PDF files. It can be used to extract text, merge and split PDFs, and encrypt and decrypt PDFs. It is a very popular library and has been around for a long time.
- pdfplumber: This is a library that allows for extracting tables and text from PDFs, it can also extract images and shapes.
- PyMuPDF: I have saved the best free python pdf library for last! With PyMuPDF not only can you access files with extensions like “.pdf”, “.xps”, “.oxps”, “.cbz”, “.fb2”, “.mobi” or “.epub” but also, about 10 popular image formats can be opened and handled like documents
Depending on the specific use-case and the complexity of the task, the best library to use may vary. IronPDF is a great choice for developers and organizations. PyPDF4 is a good choice for simple tasks such as merging and splitting PDFs.
It’s also important to note that some libraries like tabula-py and pdfplumber are focused on table extraction, other libraries like pdfquery focus on more advanced tasks like querying the PDF structure.
IronPDF – How To Process PDF Files With IronPDF?
- PDF Generation: IronPDF can create PDFs from various sources, such as URLs, HTML, Markdown, and even images. This flexibility means you can turn a wide range of content into professional-looking PDFs.
- Advanced Formatting: With IronPDF, you’re not limited to plain PDFs. You can improve your documents by adding HTML, JavaScript, custom fonts, headers, footers, and custom margins.
- PDF Editing: With IronPDF You can join, split, merge, add watermarks, and attach files to PDF pages. It also offers features for adding signatures and password protection to your PDFs, ensuring your documents are secure.
- Text and Image Extraction: IronPDF’s text and image extraction capabilities are really good. You can easily convert PDF files to text or images.
If you are a developer or part of an organization, IronPDF’s robustness, reliability, and user-friendly interface make it an excellent choice for all your PDF needs.
Let’s see how we can use it now.
First, make sure you install the .NET 6.0 SDK on your machine. Then, you will need to install the IronPDF library by running the following command:
pip install ironpdf
# Import statement for IronPDF Python
from ironpdf import *
# Use your license key
License.LicenseKey = "xxxxxxxxxxxxxxxxxxxxxxx"
To Create PDF files from HTML and URLs
# Instantiate Renderer
renderer = ChromePdfRenderer()
# Create a PDF from HTML
html = """
<h1 style='color:green'>Pythonology</h1>
<h2><a href='https://pythonology.eu'> All about Python</a></h2>
"""
Pdf_html = renderer.RenderHtmlAsPdf(html)
# Export to a file
Pdf_html.SaveAs("html_to_pdf.pdf")
# Create a PDF from a URL
Pdf_url = renderer.RenderUrlAsPdf("https://pythonology.eu/what-is-the-best-python-pdf-library/")
# Export to a file
Pdf_url.SaveAs("url_to_pdf.pdf")
The above code creates an instance of the ChromePdfRenderer, which is responsible for converting HTML content and web pages to PDF format. Then we call RenderHtmlAsPdf() method on the renderer object and pass the HTML string. This generates a PDF document and we save the output file using the SaveAs() method.
Similarly, we call the RenderUrlAsPdf() method on the renderer object and pass the URL of the web page, which helps render the HTML content from the URL and convert it into a PDF document.
If you want to add watermark to your PDF files
# Let's add watermark to the second pdf we created
Pdf_url.ApplyWatermark("<h2 style='color:red; font-size:100px' >Pythonology</h2>",10, VerticalAlignment.Middle,HorizontalAlignment.Center)
# make sure to save it
Pdf_url.SaveAs("watermarked.pdf")
OUTPUT PDF File
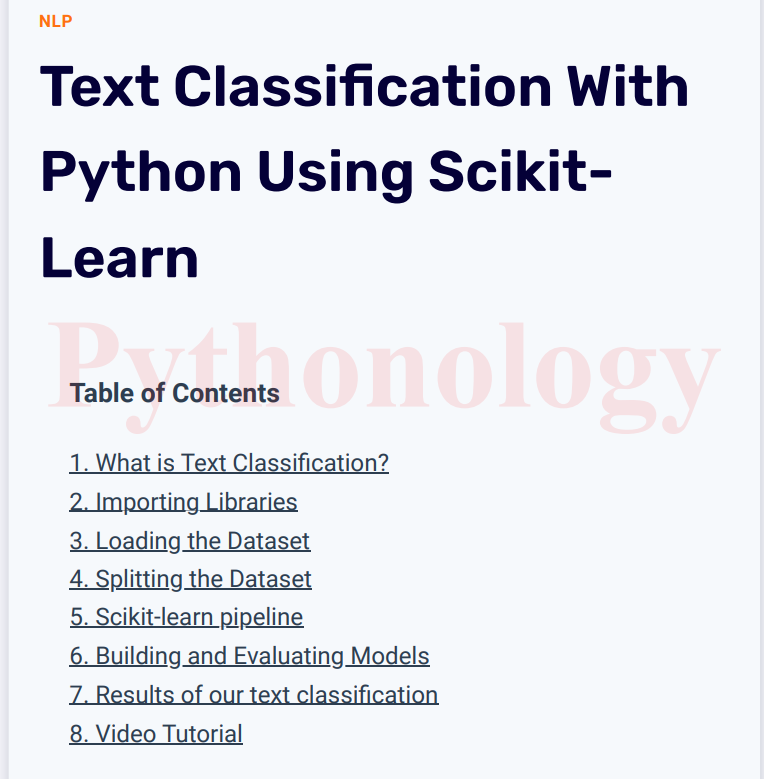
Now, let’s extract text from PDF documents
# Load existing PDF document
pdf = PdfDocument.FromFile("content.pdf")
# Extract text from PDF document
all_text = pdf.ExtractAllText()
# Extract text from specific page in the document
page_2_text = pdf.ExtractTextFromPage(1)
Take a look at their pricing and try it first before purchase. They also offer a free 30-days trial license. Check out the IronPDF’s tutorial page for a detailed explanation.
PyPDF: how to process pdf files with PyPDF?
This is a pure Python library that can be used to read and write PDF files. It can be used to extract document information, merge and split PDFs, and encrypt and decrypt PDFs. It is a very popular library and has been around for a long time.
First, you will need to install the PyPDF library by running the following command:
pip install pypdf
- Next, you can use the following code to read the contents of a PDF file:
from pypdf import PdfReader
reader = PdfReader("file.pdf")
# Print the number of pages in the PDF
print(f"There are {len(reader.pages)} Pages")
# Get the first page (index 0)
page = reader.pages[0]
# Use extract_text() to get the text of the page
print(page.extract_text())
# Go through every page and get the text
for i in range(len(reader.pages)):
page = reader.pages[i]
print(page.extract_text())
The above code is a simple example of how to read the contents of a PDF file. You can also use PyPDF to merge, split, and encrypt PDFs, as well as extract metadata and annotations.
Here is the code to extract the images from a PDF file using PyPDF:
from pypdf import PdfReader
reader = PdfReader("file.pdf")
for img in page.images:
with open(img.name, "wb") as fp:
fp.write(img.data)
Other libraries like pdfminer and slate are also popular for more advanced PDF processing tasks like extracting tables and images.
Subscribe to Receive the Latest Python Tips!
pdfplumber: how to process pdf files with pdfplumber?
pdfplumber is a Python library for pdf processing that allows for extracting text, images, and tables from PDF files. Here is an example of how you can use pdfplumber to extract text from a PDF file:
- First, you will need to install the pdfplumber library by running the following command:
pip install pdfplumber
- Next, you can use the following code to extract text from a PDF file:
import pdfplumber
with pdfplumber.open('example.pdf') as pdf:
# iterate over each page
for page in pdf.pages:
# extract text
text = page.extract_text()
print(text)
The above code uses the pdfplumber.open() function to open the PDF file, and the .pages attribute to access each page. The extract_text() method is used to extract the text from each page.
pdfplumber also allows you to extract tables from pdfs. You can use extract_tables() method to extract all the images from the PDF.
Here’s an example of how you can use pdfplumber to extract tables from a PDF file:
import pdfplumber
with pdfplumber.open('file.pdf') as pdf:
# iterate over each page
for page in pdf.pages:
print(page.extract_tables())
This code uses the pdfplumber.open() function to open the PDF file, and the .pages attribute to access each page. The code then iterates over the pages and extracts each table.
PyMuPdf: how to process pdf files with PyMuPdf?
PyMuPDF library is a Python library that allows you to extract text, images, links from PDF files. You can also convert the pages into images as shown in the example code below.
With PyMuPDF you can access files with extensions like “.pdf”, “.xps”, “.oxps”, “.cbz”, “.fb2”, “.mobi” or “.epub”. In addition, about 10 popular image formats can also be opened and handled like documents.
Here is an example of how you can use PyMuPDF to extract text, and links from a PDF file or convert Pdf to image:
- First, you will need to install the PyMuPDF library by running the following command:
pip install --upgrade pymupdf
- Next, you can use the following code to extract text, images, links from a PDF file:
# The import name for this library is fitz
import fitz
# Create a document object
doc = fitz.open('file.pdf') # or fitz.Document(filename)
# Extract the number of pages (int)
print(doc.page_count)
# the metadata (dict) e.g., the author,...
print(doc.metadata)
# Get the page by their index
page = doc.load_page(0)
# or page = doc[0]
# read a Page
text = page.get_text()
print(text)
# Render and save the page as an image
pix = page.get_pixmap()
pix.save(f"page-{page.number}.png")
# get all links on a page
links = page.get_links()
print(links)
# Render and save all the pages as images
for i in range(doc.page_count):
page = doc.load_page(i)
pix = page.get_pixmap()
pix.save("page-%i.png" % page.number)
# get the links on all pages
for i in range(doc.page_count):
page = doc.load_page(i)
link = page.get_links()
print(link)
For a more detailed explanation refer to the PyMuPDF’s official tutorial page.
Conclusion
People who do text analytics often find themselves working with PDF files and in order to extract data to process it using text analytics tools, you need to convert it to other formats such as the text format. The Python PDF libraries introduced above can help you with that. Try them and see which one is best for your project.
IronPDF offers the most features and if you need some advanced features, then it is worth trying it out. If you are looking for a free pdf library and are working with a small number of PDF files and do not need that many features, then PyMuPDF library can be a great free choice.
P.S. If you like this content, you may want to check out our youtube channel called pythonology and subscribe to our newsletter.
The video tutorial for pdf processing with python
Did you learn something from the post? Subscribe to the newsletter to learn more Python tips and tricks!